Getting Started
Picking Orders from Channels
In Omnitron, channel-related operations are carried out in the channels module, and integrations related to channels are done in the integration module. Under the Omnitron > Channels > Integration folder, there is a cron task for each channel that runs regularly in its own module, takes orders from channels and reflects them to Omnitron.
The operating structure of this cron task is not the same for every channel. The old cron task obtains the IDs of all active channels in the specified channel type, receives orders by querying the channel for orders with the “orders waiting for order status” filter for each channel through the service of the relevant channel, and creates orders in Omnitron with each subsequent order information.
Implemented a command model for integration in new cron tasks An integration object is created from the CommandIntegration class and the action required to pick orders is called by triggering the do_action method of this integration object.
First Method
There are certain methods allowed for each channel in the channel service. These are defined in the dictionary object channel_allowed_methods
in the service class. In order for the orders to be picked from the channel, the get_class method
must be available for calling in the relevant channel. After this control is provided, the Integration object that will enable the Omnitron to communicate with the channel is created, the login method of this object is called, and a connection is established by logging in the channel. If the connection with the channel cannot be established, the IntegrationUnauthorizedException
error is thrown. When the connection is established, the get_class
method of the Integration object is called and a dynamic query is created with the filters provided as parameters for each action defined in the actions list and the Serializer class related to the action, and a GET
query is made to the channel. In case of a successful response to the query, the transaction defined for the action is performed by calling the request_response
method. The creation of orders received from channels is also done with the create_order method
in the Integration class.
Integration
Path:omnitron.channels.integrations.channel_name.integration.Integration
Allows the Omnitron to communicate with channels. Each channel has its own Integration class.
- login(): Logs into the channel using the configuration data of the Integration class and updates the auth_token parameter of the channel object with the one from the new session.
- response_process(response, channel_id, obj, integration_obj, serializer, action_name): Defines and performs what will be done when a successful request is made for a certain method type and a certain action name with the data received following a successful request to the channel.
GET, POST, PATCH, PUT
andDELETE
methods are defined. When another method is used, an IntegrationNotDefineResponseProcess error is thrown. If the method type isGET
andaction_name
is “orders,” it calls thecreate_order
method for each order data in the query result. - create_order(data): Ensures the control of the customer, shipping address, billing address,
order_item
, transaction, and discount item data related to the order. Updates the related objects in the Omnitron; otherwise, it creates a new object. If there are separate shipping charges or discounts, it allocates them to each order item using theOrderService
andOrderItemService
services and updates the total pricing. If there is a product with a differentdata_source
, it performs the same order creation transactions on the vendor side usingVendorOrderService
. Sends the updated order information to the relevant channel. Sends the order information to the cargo company. Returns the created order. - create_orderitem(order_pk, data): Gets the product, stock, extra stock and order item image information related to the order item. After verifying the data with
OrderItemSerializer
, it updates the order item with the given datausingOrderItemService
; if not applicable, it creates a new order item object withorder_pk
. If there is no integration action object/record that keeps the information about the transaction related to this order item, it creates one or updates any existing ones usingIntegrationActionService
.create_transaction
(order, data): Gets thePOS
and mainPOS
information related to the order transaction, and updates it if there is a transaction record that matches the provided data by usingTransactionService
; otherwise, it creates a new one. UsingIntegrationActionService
, it creates an integration action object/record for keeping transaction-related information.
ReadOrderSerializer
Path:
omnitron.channels.integrations.channel_name.serializers.ReadOrderSerializer
- get_endpoint(): In the request created to query the channel, the endpoint subject to request is obtained with this method.
ChannelService
Path: omnitron.channels.service.ChannelService
The service for channel-related operations. Operations such as creating and updating channels, sending information from Omnitron to the channel or fetching up-to-date information from the channel to Omnitron are performed in this service. The get_class
method of this service is used when fetching orders from channels to Omnitron.
- get_class(channel, class_names, timeout, remote_id, filter_params): It enables Omnitron to query the classes specified in the class_names list from the channel provided as a parameter by using the Integration class of the channel. The details of the query made to the classes and the operations to be performed following the query take place in the Integration class.
Second Method
As in the first method, there is a cron task that runs at regular intervals for fetching order-related information from the channels. Although the method defined for fetching orders is implemented differently in each channel, the get_order method is generally called for each channel of that channel type in this cron task. In this method, parameters such as filtering are defined on how to fetch orders from that channel and the get_orders
method is called with these additional parameters over the integration object. The action of fetching orders from the relevant channel is performed in the channel’s integration class.
Fetching of orders may be customized based on the channel and the relevant method, such as:
- Fetching orders between the desired start and end date upon the providing of date parameters such as
start_date, end_date
andcreated_before
- Fetching specific orders using
order_ids
created in the channel withorder_numbers_to_fetch
fetching orders created after this order by providing anorder_id
- Fetching all orders regardless of order status with the
fetch_all_orders
parameter.
CommandIntegration
Path: omnitron.channels.integrations.channel_name.integration.CommandIntegration
Similar to the Integration channel, it allows the Omnitron to communicate with channels. It is inherited from the BasicIntegration and BaseIntegration classes. Since the Integration class is inherited from the BaseIntegration class, it covers what is described in the Integration section. Apart from the Integration class, it also inherits the BasicIntegration class, so various actions can be easily implemented by implementing the Command design pattern with the do_action method. This section will be detailed in the Integration document.
- do_action(key, objects=None, force=False, kwargs): With the key parameter, it obtains the serializer class for which action to take. The parameters or verification methods that each channel wants to receive upon order requests may differ, which is why each Integration class can use its own Serializer classes. The Serializer class to be used for the get_orders action is defined in the serializer_class dictionary of each channel’s Integration class. After receiving the Serializer class, it calls the
get_objects
method and then calls theqs__action_name
method for the action provided in the key. This method returns a Django ORM Query object created for how to fetch orders. An object is derived from the Serializer class to obtain the objects fetched upon query as parameters, and by calling the run method of this object, the processes of sending a request to the channel and the creation of orders by Omnitron according to the result returned upon the request are performed in the action class.
Serializer/Command
Path: omnitron.channels.integrations.channel_name.commands.GetOrders
The process of fetching and verifying the features of the order takes place in the Serializer class. In addition, as a result of the implementation of the Command design pattern, sending a request to the channel described in the first method and creating records such as order and order item, transaction etc. take place in this class. Since the "create" operations are performed as in the first method, the details will not be repeated. run(): The method that distinguishes this class from the standard Serializer classes and implements the command design pattern. Similar to the operating principle of the request_process
and response_process
methods mentioned in the first method, it creates the request to be sent to the channel with the send method, sends it and upon a successful response, it completes the action by providing the parameter the method defined for the relevant action.
Service
OrderService
Path: omnitron.orders.service.OrderService
It is the service where operations such as creating, updating, deleting the order, as well as reflecting the discount items on the products in the order and canceling the order are carried out.
- create_order(number, channel, kwargs): Checks if there is an order object with the provided number and throws an
OrderDuplicatedFieldException
if applicable; otherwise, it creates a new order object with the number in the provided channel. - update_order(order, audit_context, kwargs): Updates the placed order object and order items with the information in kwargs.
- change_billing_address(order, address): Changes the billing address of the order by using the relevant strategy.
- change_shipping_address(order, address): Changes the shipping address of the order using the relevant strategy.
- change_order_status(order, status): Changes order and item status.
- set_distribute_interest_amount(order): If there are discounts to be applied on the order items, it provides how these will be applied and recalculation of the shipping fee, applies the discount rates on the order items and updates the shipping fee of the order. If there is another discount in the basket, the discount is distributed among the products. If the discount amount is higher than the value of the product, it is distributed to other products.
- cancel_order(order, reasons, audit_context, is_all, cancel_items, forced_refund_amount, is_cargo_refund, refund_invoice_number, next_status, ibans, holder_names, diagnose): Performs the cancellation of the order and the operations related to the order item affected by the cancellation, discount rates update, refund transactions, cancellation strategy etc.
- update_cancellation_plan(cancellation_plan, status, audit_context, kwargs):** Updates the provided cancellation plan object with the data in kwargs.
- order_status_update(order): Allows the order status to be updated according to the lowest status among the active order items.
- reject_order_cancel(order, audit_context): Updates the statuses of order and items to be canceled to their previous status, and the cancellation status to "rejected." Updates the status of the cancellation request and plan of the order and items.
approved_order_cancel
(order, invoice_number, audit_context): Performs the operations related to the cancellation and refund of the orders with approved status and received payments. - get_payment_history(order, kwargs):** Fetches detailed transaction information about the order from the relevant pos gateway.
- get_transaction_amount(order): Using the
get_payment_history
method, the total amount is calculated by fetching the order-related transactions.update_cancel_status
(order, order_items, cancel_status, audit_context, kwargs): If there are order and item transactions such as cancellation plan and manual return according to the request, the controls are made and a transaction is created. Status updates are made for the order, items, cancellation plan, and cancellation request. - progress_cancel_status(payment_type, update_cancel_status):
payment_type
orders with payment methods provided as parameters are fetched, and the status of the cancellation plan objects of the order is updated to theupdate_cancel_status
provided as a parameter. - confirm_pay_on_delivery(order): It is checked whether the payment method of the order is
pay on delivery
, the order, the order items and, if any, the status of the vendor order are updated fromconfirmation waiting
toapproved
. - accept_in_store(order): Confirms that the order can be picked up from the store.
- deliver_in_store(order): Confirms that the order has been picked up from the store.
- mark_as_not_sent(order): Updates and stores the
is_sent
information that the order has been approved, but has not been sent as "False." - bulk_tracking_number_update(uploaded_file): Provides bulk tracking number update for all orders in the file provided as a parameter.
OrderItemService
Path: omnitron.orders.service.OrderItemService
Where the transactions related to the products in the order are made.
- create_order_item(order, product, image, kwargs): An order item is created for the order object and product provided as a parameter. It updates the stock information of the order item using the product stock service.
- update_order_item(order_item, image, shipping_update, force, audit_context, kwargs): Updates the order item provided as a parameter with the information sent with kwargs and the image parameter.
- update_order_item_send_stock(order_item): Updates the stock information of the product for the placed order item.
- send_shipping_info(order_items): If there are vendor order items for the ordered items, the vendor sends the shipping information of the order item to the relevant data source, and updates the order item according to this information.
Model
Structure that represents the tables in the database.
Order
Path: omnitron.orders.models.Order
The Order Model is inherited from the OmnitronBaseOrder
model. The OmnitronBaseOrder
model is inherited from the omnicore.orders.models.BaseOrder
model.
OmnitronBaseOrder
Name | Description |
Number | CharField, every order has an order number. |
Status | EnumField; stores the status of the order item. It can take values from the omnicore.orders.enums.OrderStatus model. |
date_placed | DateTimeField, date placed |
extra_field | JSONField |
delivery_type | EnumField, ShippingOptionDeliveryType |
checkout_provider_id | IntegerField, provider id |
e_archive_url | URLField, E-Archive address |
remote_addr | GenericIPAddressField |
Shipping_address | ForeignKey, ID of the shipping address |
Billing_address | ForeignKey, ID of the billing address |
Currency | EnumField, stores the currency of the order item fee. Gets the values in the omnicore.catalogs.enums.CurrencyType class. These may be tr, eu, usd, egp, gbp, mad, pln, sar, ron, uah, czk, huf, rub, bgn and iqd. This is TR by default. |
Amount | DecimalField, order amount |
Discount_amount | DecimalField, discount amount |
Shipping_amount | DecimalField, shipping amount |
Shipping_tax_rate | DecimalField, shipping tax rate |
Refund_amount | DecimalField, refund amount |
Discount_refund_amount | DecimalField, discount refund amount |
Shipping_refund_amount | DecimalField, shipping refund amount |
Invoice_number | CharField, invoice number |
Invoice_date | DateTimeField, invoice date |
Tracking_number | CharField, tracking number |
Shipping_company | EnumField, stores the information of the shipping company. May get the information defined in the omnicore.orders.enums. ShippingCompany class. |
Channel | ForeignKey, ID of the order channel |
Customer | ForeignKey, ID of the customer placing the order |
Payment_option | ForeignKey, ID of the payment option |
Payment_option_slug | SlugField, slug code of the payment option |
Bin_number | CharField, a field regarding the bank information related to the payment. |
Installment | ForeignKey, related to installments |
Installment_count | PositiveIntegerField, installment count |
Installment_interest_amount | DecimalField, installment interest amount |
Cargo_company | ForeignKey, ID of the cargo company |
Is_send | BooleanField, stores the information whether the order has been sent. |
Cancellation_info | JSONField, stores cancellation information. |
Cancel_status | EnumField, stores cancel status. May get the values in the CancelStatus model. These are respectively:
|
Shipping_interest_amount | DecimalField, shipping interest amount |
External_status | ForeignKey, stores different displays of order statuses in applications such as OMS or on the market side. |
ClientType | EnumField, stores the default client type. The client can be Android, iOS, In-Store or default. |
Carrier_shipping_code | CharField, carrier shipping code |
Segment | CharField |
has_gift_box | NullBooleanField, has gift box |
gift_bpx_note | CharField, gift box note |
language_code | CharField, language code |
notes | CharField, instructions |
delivery_range | DateTimeRangeField |
shipping_option_slug | SlugField, shipping option slug |
Relationship Between Models

Figure 1: Associated Order Model
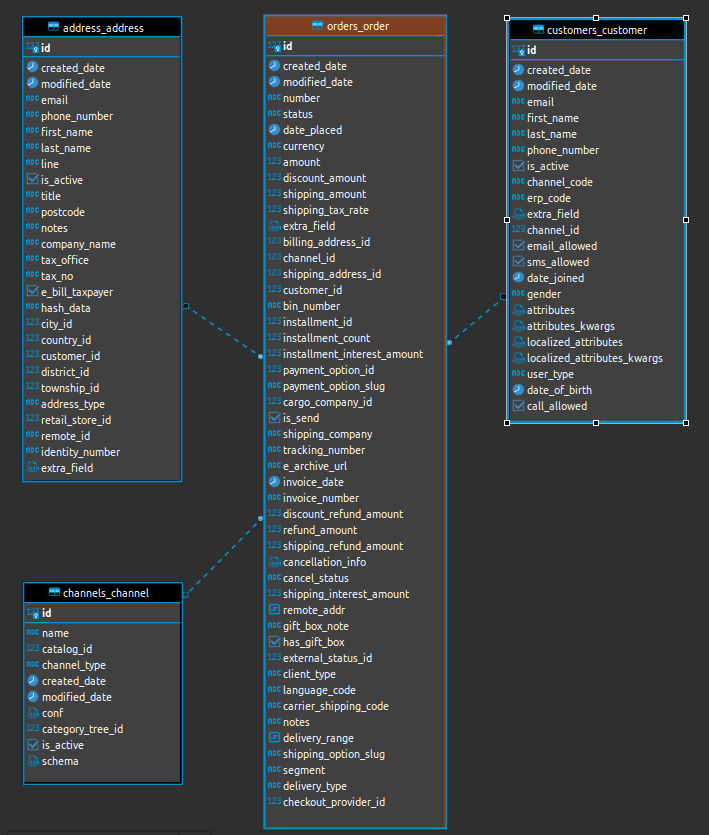
Figure 2: Associated Channel, Address and Customer Models
OrderItem
Path: omnitron.orders.models.OrderItem
Inherited from the OmnitronBaseOrderItem
model. And this model is inherited from the omnicore.orders.models.BaseOrderItem
model.
Name | Description |
Order | ForeignKey, order ID of the order item |
Product | ForeignKey, product ID of the order item |
Status | EnumField; stores the status of the order item. It can take values from the omnicore.orders.enums.OrderStatus model. These are:
|
attributes | JsonField, product features |
attributes_kwargs | JsonField, key of product features |
localized_attributes | JsonField, localized product features |
localized_attributes_kwargs | JsonField, key of localized product features |
extra_field | JsonField |
e_archive_url | URLField, E-Archive address |
Price_currency | EnumField, stores the currency of the order item fee. Gets the values in the omnicore.catalogs.enums.CurrencyType class. These may be tr, eu, usd, egp, gbp, mad, pln, sar, ron, uah, czk, huf, rub, bgn and iqd. |
Price | DecimalField, price of the order item |
Tax_rate | DecimalField, tax rate |
Invoice_number | CharField, invoice number |
Invoice_date | DateTimeField, invoice date |
Tracking_number | CharField, tracking number |
Shipping_company | EnumField, stores the information of the shipping company. May get the information defined in the omnicore.orders.enums.ShippingCompany class. |
Retail_price | DecimalField, retail price |
Image | ImageField, order item image |
Parent | ForeingKey(’self’), ID of an inherited order item |
Estimated_delivery_date | DateField, estimated delivery date |
Price_list | ForeignKey, ID of the price list for the order item |
Stock_list | ForeignKey, ID of the stock list for the order item |
Discount_amount | DecimalField, discount amount |
External_status | ForeignKey, stores the status of the order item on platforms such as OMS |
Shipped_date | DateTimeField, shipped date of the order item |
Delivered_date | DateTimeField, delivered date of the order item |
Carrier_shipping_code | CharField, carrier shipping code |
Transactions | ManyToManyField, stores payment transactions related to the order item. |
Funds_transactions | ManyToManyField, stores fund transactions related to the order item. |
Payondelivery_transactions | ManyToManyField, stores pay-on-delivery transactions related to the order item. |
Loyalty_transactions | ManyToManyField, stores loyalty transactions related to the order item. |
Cashregister_Transactions | ManyToManyField, stores cash register transactions related to the order item. |
Reconciliation | ForeignKey, ID of the reconciliation for the order item. |
Cancellation_reconciliation | ForeignKey, ID of the cancellation reconciliation for the order item. |
Cancel_status | EnumField, stores cancel status. May get the values in the CancelStatus model. These are respectively:
|
Forced_refund | BooleanField, stores whether there is a forced refund. |
Installment_interest_amount | DecimalField, installment interest amount for the order item. |
Discount_amount | DecimalField, discount amount for the order item. |
datasource | ForeignKey, ID of the channels.DataSource model. |
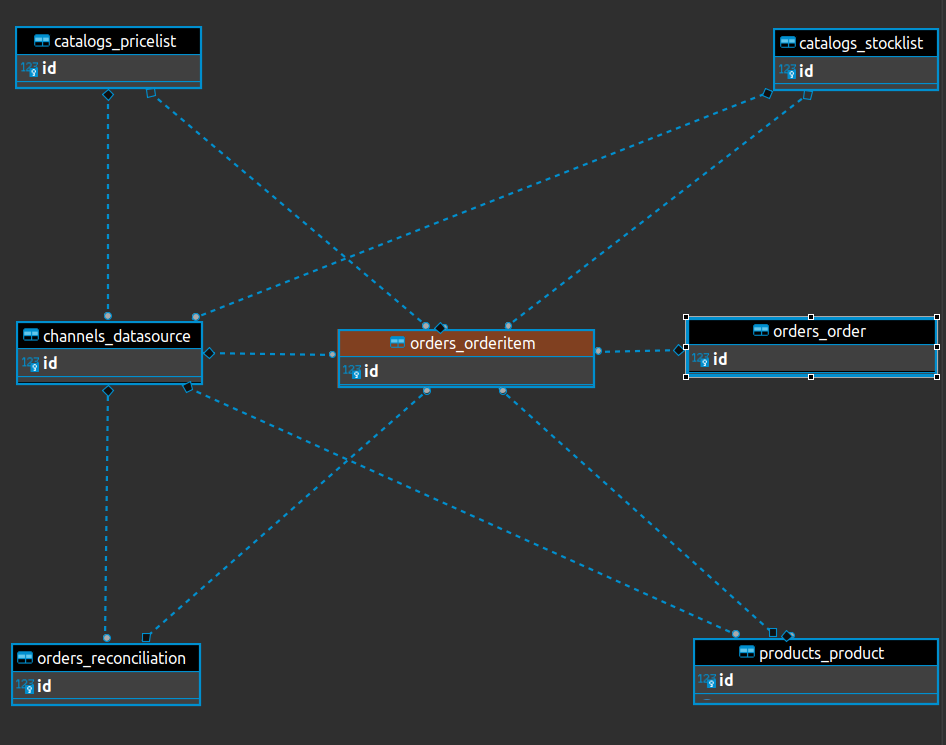
Figure 3: Associated Models with OrderItem
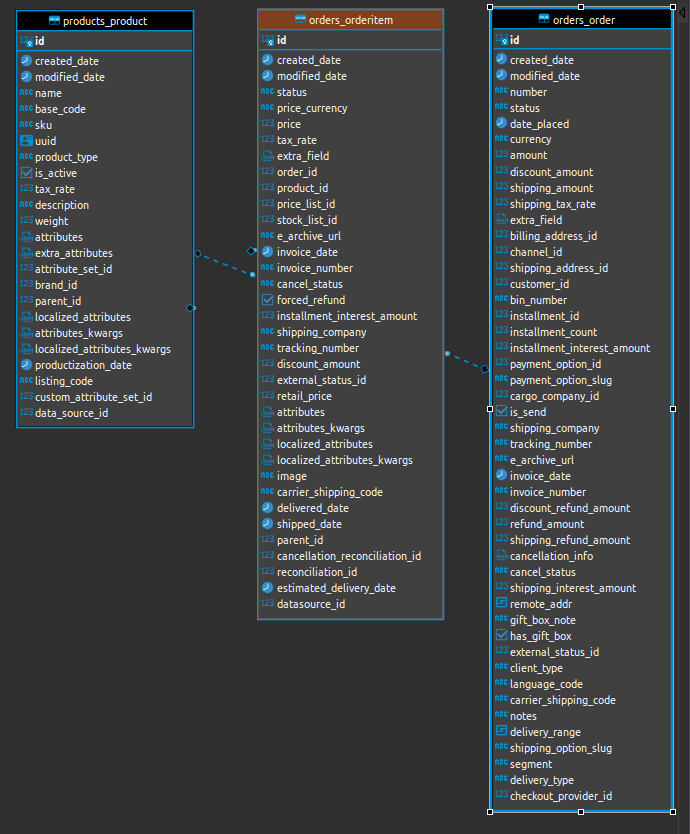
Figure 4: Product and Order Models Related to OrderItem
ViewSet
The part where the endpoints are connected to each other and the controls and processing are made for the transactions to be performed by the service.
OrderViewSet
omnitron.orders.resources.views.OrderViewSet
Path: /api/v1/orders/
GET, POST, PATCH, PUT
and DELETE
requests are allowed. Like other viewsets, create, update and destroy are performed through the service. Details of the aforementioned Service are available.
Custom endpoints:
POST
change_billing_addressPOST
change_shipping_addressPATCH
change_order_statusPOST
create_mappingPOST
cancelPOST
cancellation_reject_orderPOST
cancellation_approved_orderPOST
update_cance_statusPOST
accept_in_storePOST
deliver_in_storeGET
update_order_statusGET
get_remote_idPOST
mark_as_not_sentPOST
split_packagesPOST
bulk_tracking_number_updateGET
bulk_tracking_number_update_status
The structure of these endpoints will be shown with an example, as they return the serialized order after sending the request and the data in the request to the service and performing the operation in the service, and these operations are detailed in the Service section.
GET
All Orders
Endpoint that allows all orders to be retrieved in paginated form.
Path: api/v1/orders/
Response
The link and details of the orders required to reach the next page, the total number of orders, are sent as a response.
{
"count": 101,
"next": "{customer_omnitron_url}/api/v1/orders/?page=2",
"previous": null,
"results": [
{
"pk": 34,
"number": "7cf135a8056542e",
"channel": 1,
"status": "400",
"date_placed": "2017-02-02T16:04:07.574254Z",
"customer": 1106,
"shipping_address": 30,
"billing_address": 30,
"currency": "try",
"amount": "19.62",
"shipping_amount": "8.50",
"shipping_tax_rate": null,
"extra_field": {
"cargo_line_id": "6d53c809-c55c-49e6-8e88-a70f00ef9d01"
},
"payment_option": 2,
"payment_option_slug": "credit_card",
"bin_number": "454360",
"installment": 22,
"installment_count": 1,
"installment_interest_amount": "0.00",
"cargo_company": 1,
"invoice_number": null,
"invoice_date": null,
"e_archive_url": null,
"refund_amount": "0.00",
"discount_refund_amount": "0.00",
"shipping_refund_amount": "0.00",
"discount_amount": "0.00",
"is_send": true,
"net_shipping_amount": 8.5,
"shipping_interest_amount": "0.00",
"tracking_number": null,
"carrier_shipping_code": null,
"remote_addr": null,
"fundstransfertransaction_set": [],
"has_gift_box": false,
"gift_box_note": null,
"external_status": null,
"client_type": "default",
"language_code": null,
"notes": null,
"delivery_range": null,
"shipping_option_slug": null,
"segment": null
}
]
}
GET
Order By Order Number
Following method is used to get information of all related orders including {number} value in any part of the order number.
Path: /api/v1/orders/?number={number}
Following method is used to ger information of a single order in which the order number is exactly same as the {number}.
Path: /api/v1/orders/?number__exact={number}
Response
The detailed information of the order belonging to the order number specified in the request is sent as a response.
Example Request: /api/v1/orders/?number=8610
{
"count": 2,
"next": null,
"previous": null,
"results": [
{
"pk": 277,
"number": "1222078610011628",
"channel": 1,
"status": "400",
"date_placed": "2019-12-27T07:00:21.457087Z",
"customer": 1867,
"shipping_address": 1875,
"billing_address": 1875,
"currency": "try",
"amount": "200.00",
"shipping_amount": "0.00",
"shipping_tax_rate": null,
"extra_field": {},
"payment_option": 6,
"payment_option_slug": "mobilexpress",
"bin_number": null,
"installment": 3,
"installment_count": 1,
"installment_interest_amount": "0.00",
"cargo_company": 1,
"invoice_number": null,
"invoice_date": null,
"e_archive_url": null,
"refund_amount": "100.00",
"discount_refund_amount": "0.00",
"shipping_refund_amount": "0.00",
"discount_amount": "0.00",
"is_send": true,
"net_shipping_amount": 0,
"shipping_interest_amount": "0.00",
"tracking_number": null,
"carrier_shipping_code": null,
"remote_addr": "213.194.76.106",
"fundstransfertransaction_set": [],
"has_gift_box": false,
"gift_box_note": null,
"external_status": null,
"client_type": "default",
"language_code": "tr-tr",
"notes": null,
"delivery_range": null,
"shipping_option_slug": null,
"segment": null,
"modified_date": "2021-09-15T11:07:47.931518Z"
},
{
"pk": 182,
"number": "1186104634112903",
"channel": 1,
"status": "550",
"date_placed": "2019-11-15T15:41:27.849836Z",
"customer": 24,
"shipping_address": 25,
"billing_address": 25,
"currency": "try",
"amount": "107.00",
"shipping_amount": "7.00",
"shipping_tax_rate": null,
"extra_field": {},
"payment_option": 2,
"payment_option_slug": "credit_card",
"bin_number": "555555",
"installment": 3,
"installment_count": 1,
"installment_interest_amount": "0.00",
"cargo_company": 1,
"invoice_number": null,
"invoice_date": null,
"e_archive_url": null,
"refund_amount": "0.00",
"discount_refund_amount": "0.00",
"shipping_refund_amount": "0.00",
"discount_amount": "0.00",
"is_send": true,
"net_shipping_amount": 7,
"shipping_interest_amount": "0.00",
"tracking_number": "19025",
"carrier_shipping_code": null,
"remote_addr": "213.194.76.106",
"fundstransfertransaction_set": [],
"has_gift_box": false,
"gift_box_note": null,
"external_status": null,
"client_type": "default",
"language_code": "tr-tr",
"notes": null,
"delivery_range": null,
"shipping_option_slug": null,
"segment": null,
"modified_date": "2021-09-10T03:51:41.052320Z"
}
]
}
POST
Update Cancel Status
Path: /api/v1/orders/151/update_cancel_status/
Endpoint that is used to change the cancellation status of the order.
{
"order": "<order_id>",
"order_items": [order_item1_id, order_item2_id],
"cancel_status": "cancel_status"
}
Response
According to the data transmitted in the request, the order whose cancel_status field has been updated and all data including the order items belonging to this order will be sent as a response. Properties are in Python format.
{
"pk": 111,
"number": "1535788520612146",
"channel": " ",
"status": "400",
"date_placed": "2020-12-24T09:07:54.639779Z",
"customer": " ",
"shipping_address": "{..}",
"billing_address": "{...}",
"currency": "try",
"amount": "336.38",
"shipping_amount": "8.50",
"shipping_tax_rate": null,
"extra_field": {},
"orderitem_set": [
{
"pk": 221,
"order": 111,
"product": "{...}",
"status": "400",
"price_currency": "try",
"price": "127.98",
"tax_rate": "8.00",
"extra_field": "{...}",
"price_list": "{...}",
"stock_list": "{...}",
"invoice_number": null,
"invoice_date": null,
"e_archive_url": null,
"cancel_status": "manuel_refund_need",
"status_display": "approved",
"installment_interest_amount": "0.00",
"net_amount": "127.98",
"tracking_number": null,
"shipping_company": null,
"discount_amount": "0.00",
"shipment_code": null,
"benefitapplicant_set": [],
"external_status": null,
"retail_price": "319.96",
"attributes": {},
"attributes_kwargs": {},
"tracking_url": null,
"image": null,
"parent": null,
"data_source": null,
"estimated_delivery_date": null
}
],
"discountitem_set": [],
"transaction_set": [],
"payment_option": "{...}",
"payment_option_slug": "credit_card",
"bin_number": null,
"installment": "{...}",
"installment_count": 1,
"installment_interest_amount": "0.00",
"cargo_company": "{...}",
"invoice_number": null,
"invoice_date": null,
"e_archive_url": null,
"refund_amount": "0.00",
"discount_refund_amount": "0.00",
"shipping_refund_amount": "0.00",
"discount_amount": "0.00",
"cancellation_info": "{...}",
"status_display": "approved",
"tracking_number": null,
"is_send": true,
"cancel_status": "manuel_refund_ned",
"net_shipping_amount": 8.5,
"remote_addr": "127.0.0.1",
"language_code": "tr-tr",
"fundstransfertransaction_set": [],
"has_gift_box": false,
"gift_box_note": null,
"tracking_url": null,
"external_status": null,
"payondeliverytransaction_set": [],
"loyaltytransaction_set": [],
"bextransaction_set": [],
"cancellationplan_set": [],
"cashregistertransaction_set": [],
"client_type": "default",
"shipping_interest_amount": "0.00",
"notes": null,
"delivery_range": null,
"shipping_option_slug": "UPS",
"segment": null
}